Preventing CSRF With a Session Variable
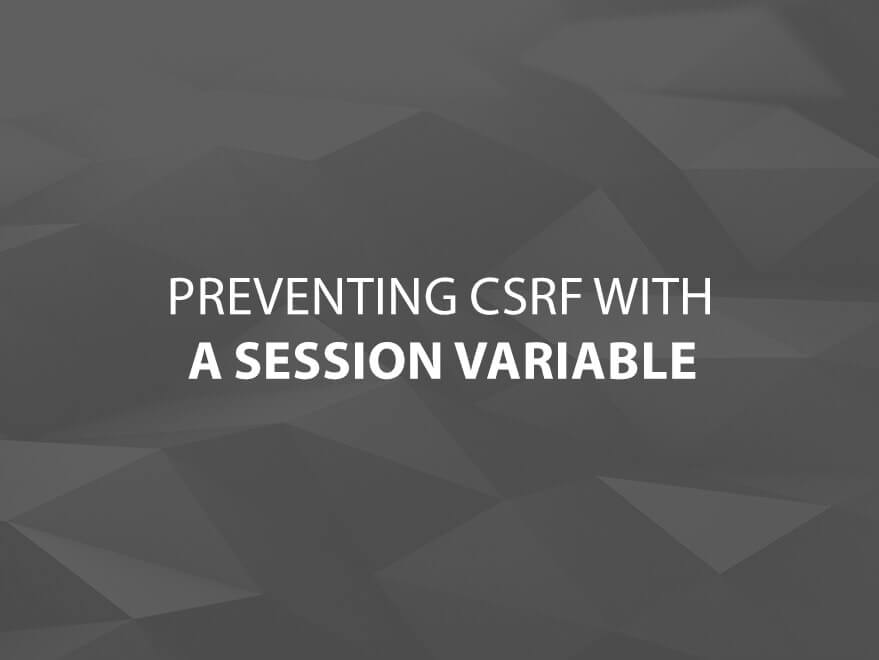
Cross Site Request Forgery (CSRF) is a growing concern for web developers as more and more viruses and malicious scripts are created in an attempt to surpass web based authentication systems. If you don’t know what CSRF is, it is a way of performing a kind of session hijacking, where a malicious pre-defined page request is sent from the user’s computer to a website that they are already logged into. This is able to bypass any server side authentication because the web server views this request as coming from the user, and therefore is a part of the session that the user has ostensibly already begun by entering their credentials. Once the request reaches the web server, there is no way of knowing whether the request was intentional, or was made with a CSRF attack.
One way to prevent CSRF is to check the request “referer” HTTP header. This is meant to ensure that the request originated from the intended source web-page. Unfortunately, this header can sometimes be manipulated by the attacker, especially if they are already sending modified post data to your server. Luckily, CSRF attacks are fairly easy to prevent using a simple server side session variable.
So how do we, as PHP developers, stop a CSRF attack? Here’s how:
When a session is started, initialize a session variable with a random number.
<?php $_SESSION[‘csrf’] = rand(); ?>
This variable will be passed as a hidden input to all post forms.
<input type=”hidden” name=”csrf” value=”<?php echo $_SESSION[‘csrf’]; ?>” />
When the page is posted, check that the value of the hidden variable is equal to the value stored in the user’s session.
<?php if($_POST[‘csrf’] == $_SESSION[‘csrf’]) { return true; } else { return false; } ?>
In order for an attacker to send a viable page request to the server, they would need to dynamically retrieve the value of this variable, something that the vast majority of CSRF attacks are simply unable to do. This will prevent all but the most complex, dynamic CSRF attack, without compromising performance, adding a huge amount of code or causing your end users to jump through any hoops.
Comments