Sending an HTTP Request in PHP Without cURL
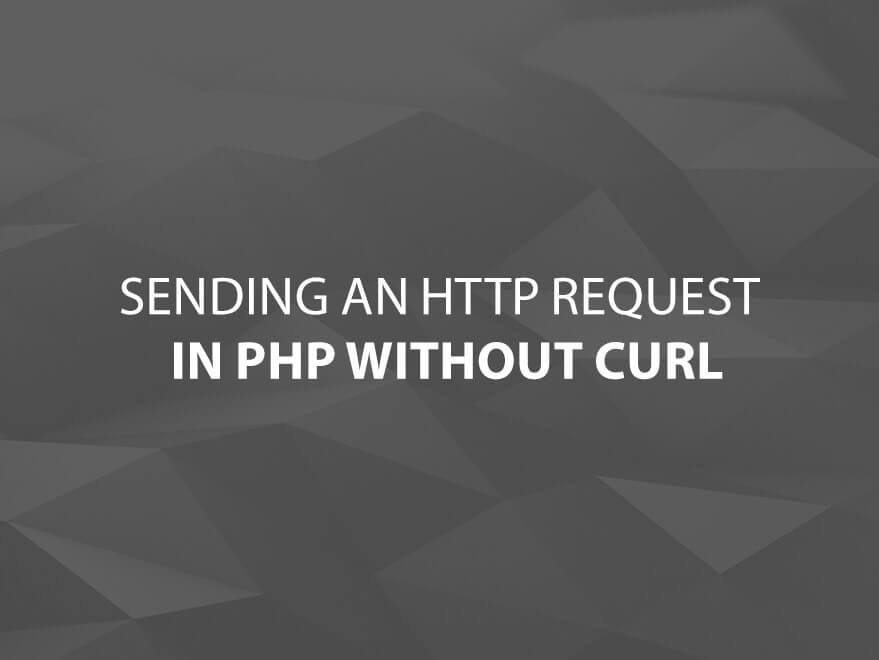
Most people who want to send page requests from PHP use the cURL library, but making sure that the cURL library is installed on the server is not always possible. You don’t need cURL to make a page request, in fact, in some cases it is just easier to use the built in functionality of PHP.
The following code is an example of a very simple page with a single text input and a PHP script that generates a valid HTTP request, sends it to the URL that the user entered, and reads the HTTP standard response code generated by the server that handled the request.
<?php if(isset($_POST['link'])) { $link = $_POST['link']; //remove protocol, we're assuming its http if(strstr($link, '://') != false){ $link = substr($link, strpos($link,'://') + strlen('://')); } //split the url into host (www.example.com) and requested page (index.php) on the first slash $host = substr($link, 0, strpos($link, '/')); $request = substr($link, strpos($link, '/')); //open a socket connection to the site on port 80 (http protocol port) $fp = @fsockopen($host, 80, $errno, $errstr); if($fp == false){ $response = $errno . " " . $errstr; } else { //create the request headers $headers = array(); $headers[] = 'HEAD ' . $request . ' HTTP/1.1'; $headers[] = 'Host: ' . $host; $headers[] = 'Content-Length: 0'; $headers[] = 'Connection: close'; $headers[] = 'Content-Type: text/html'; $headers = implode("\r\n", $headers) . "\r\n\r\n"; //send our request if (!fwrite($fp, $headers)) { fclose($fp); $response = "Could not access webpage specified."; } else { //read the response $rsp = ''; while(!feof($fp)) { $rsp .= fgets($fp,8192); } fclose($fp); $hunks = explode("\r\n\r\n",trim($rsp)); $headers = explode("\n", $hunks[count($hunks) - 1]); $response = trim($headers[0]); } } } ?> <html> <head> <title>Test Link</title> </head> <body> <?php if(isset($response)) { echo $response; } ?> <form method="post"> <label for="link">Link</label> <input id="link" type="text" name="link" /> </form> </body> </html>
Obviously this is about as simple as you can get, with a little extra code you can read back the entire page response, or add get and post variables to your request. Everything that you can do with cURL, you can also do without it.
Comments